C Programming - Arrays:
Array is a collection of same type elements under the same variable identifier referenced by index number. Arrays are widely used within programming for different purposes such as sorting, searching and etc. Arrays allow you to store a group of data of a single type. Arrays are efficient and useful for performing operations . You can use them to store a set of high scores in a video game, a 2 dimensional map layout, or store the coordinates of a multi-dimensional matrix for linear algebra calculations.
Arrays are of two types single dimension array and multi-dimension array. Each of these array type can be of either static array or dynamic array. Static arrays have their sizes declared from the start and the size cannot be changed after declaration. Dynamic arrays that allow you to dynamically change their size at runtime, but they require more advanced techniques such as pointers and memory allocation.
It helps to visualize an array as a spreadsheet. A single dimension array is represented be a single column, whereas a multiple dimensional array would span out n columns by n rows. In this tutorial, you will learn how to declare, initialize and access single and multi dimensional arrays.
Single Dimension Arrays:
Declaring Single Dimension Arrays:
Arrays can be declared using any of the data types available in C. Array size must be declared using constant value before initialization. A single dimensional array will be useful for simple grouping of data that is relatively small in size. You can declare a single dimensional array as follows:<data type> array_name[size_of_array];
Say we want to store a group of 3 possible data information that correspond to a char value, we can declare it like this:
char game_map[4];
Initializing Single Dimension Arrays:
Array can be initialized in two ways, initializing on declaration or initialized by assignment.Initializing on Declaration:
If you know the values you want in the array at declaration time, you can initialize an array as follows:
<data type> array_name[size_of_array] = {element 1,
element 2, ...};
C Programming - Pointers:
Pointers are widely used in programming; they are used to refer to memory location of another variable without using variable identifier itself. They are mainly used in linked lists and call by reference functions.Diagram 1 illustrates the idea of pointers. As you can see below; Yptr is pointing to memory address 100.
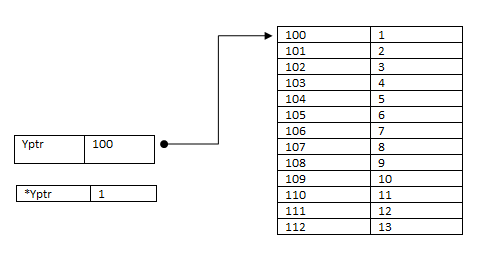
Diagram 1: 1. Pointer and memory relationship
Pointer Declaration;
Declaring pointers can be very confusing and difficult at times (working with structures and pointer to pointers). To declare pointer variable we need to use * operator (indirection/dereferencing operator) before the variable identifier and after data type. Pointer can only point to variable of the same data type.Syntax
Datatype * identifier;
Address operator:
Address operator (&) is used to get the address of the operand. For example if variable x is stored at location 100 of memory; &x will return 100.This operator is used to assign value to the pointer variable. It is important to remember that you MUST NOT use this operator for arrays, no matter what data type the array is holding. This is because array identifier (name) is pointer variable itself. When we call for ArrayA[2]; ‘ArrayA’ returns the address of first item in that array so ArrayA[2] is the same as saying ArrayA+=2; and will return the third item in that array.
Pointer arithmetic:
Pointers can be added and subtracted. However pointer arithmetic is quite meaningless unless performed on arrays. Addition and subtraction are mainly for moving forward and backward in an array.Note:
you have to be very careful NOT to exceed the array elements when you use arithmetic; otherwise you will get horrible errors such as “access violation”. This error is caused because your code is trying to access a memory location which is registered to another program.
Operator
|
Result
|
++
|
Goes to the next memory location that the pointer is pointing to.
|
--
|
Goes to the previous memory location that the pointer is pointing to.
|
-= or -
|
Subtracts value from pointer.
|
+= or +
|
Adding to the pointer
|
Pointer to Arrays:
Array identifier is a pointer itself. Therefore & notation shouldn’t be used with arrays. The example of this can be found at code 3. When working with arrays and pointers always remember the following:- Never use & for pointer variable pointing to an array.
- When passing array to function you don’t need * for your declaration.
- Be VERY CAREFUL not to exceed number of elements your array holds when using pointer arithmetic to avoid errors.
Pointers and Structures:
Pointers and structures is broad topic and it can be very complex to include it all in one single tutorial. However pointers and structures are great combinations; linked lists, stacks, queues and etc are all developed using pointers and structures in advanced systems.Pointer to Pointer:
Pointers can point to other pointers; there is no limit whatsoever on how many ‘pointer to pointer’ links you can have in your program. It is entirely up to you and your programming skills to decide how far you can go before you get confused with the links. Here we will only look at simple pointer to pointer link. Pointing to pointer can be done exactly in the same way as normal pointer. Diagram below can help you understand pointer to pointer relationship.Diagram 2. Simple pointer to pointer relationship
Code for diagram 2:
Char *ptrA;
Char x=’b’;
Char *ptrb;
ptrb=&x;
ptrA=&ptrb;
ptrA gives 100, *ptrA gives 101 , ptrb is 101 ,*ptrb gives b, **ptrA gives b
comment: **ptrA means ‘value stored at memory location of value stored in memory location stored at PtrA’
No comments:
Post a Comment