Modular programming:
A programming style that braks down program functions into modules, each of which accomplishes one function and contains all the source code and variables needed to accomplish that function. Modular programming is a solution to the problem of very large programs that are difficult to debug and maintain. By segmenting the program into modules that perform clearly defined functions, you can determine the source of program erros more easly. Object-orientated programming languages, such as SmallTalk and HyperTalk, incorporate modular programming principles.Function:
A C program has atleast one function [ main( ) ]. Without main() , there is technically no C program.
Types of C functions:
Basically, there are two types of functions in C on basis of whether it is defined by user or not.- Library function
- User defined function
Library function:
Library functions are the in-built function in C programming system. For example:main() - The execution of every C program starts from this main.
printf() - prinf() is used for displaying output in C.
scanf() - scanf() is used for taking input in C.Visit this page to learn more about library functions in C programming.
User defined function
C provides programmer to define their own function according to their requirement known as user defined functions.Example of how C function works:
#include <stdio.h>
void function_name(){
................
................
}
int main(){
...........
...........
function_name();
...........
...........
}
As mentioned earlier, every C program begins from main() and program starts executing the codes inside main function. When the control of program reaches to function_name() inside main. The control of program jumps to "void function_name()" and executes the codes inside it. When, all the codes inside that user defined function is executed, control of the program jumps to statement just below it. Analyze the figure below for understanding the concept of function in C.
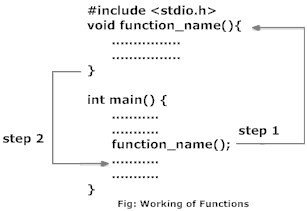
Remember, the function name is an identifier and should be unique.
Advantages of user defined functions:
- User defined functions helps to decompose the large program into small segments which makes programmar easy to understand, maintain and debug.
- If repeated code occurs in a program. Function can be used to include those codes and execute when needed by calling that function.
- Programmar working on large project can divide the workload by making different functions.
Function prototype(declaration):
Every function in C programming should be declared before they are used. These type of declaration are also called function prototype. Function prototype gives compiler information about function name, type of arguments to be passed and return type.Syntax of function prototype:
return_type function_name(type(1) argument(1),....,
type(n) argument(n));
Here, in the above example, function prototype is "int add(int a, int b);" which provides following information to the compiler:
- name of the function is "add"
- return type of the function is int.
- two arguments of type int are passed to function.
Function call:
Control of the program cannot be transferred to user-defined function (function definition) unless it is called (invoked).Syntax of function call:
function_name(argument(1),....argument(n));
In the above
example, function call is made using statement "add(num1,num2);" in line
8. This makes control of program transferred to function
declarator(line 12). In line 8, the value returned by function is kept
into "sum" which you will study in detail in function return type of
this chapter.Function definition:
Function definition contains programming codes to perform specific task.Syntax of function definition:
return_type function_name(type(1) argument(1),.
....,type(n) argument(n))
{
//body of function
}
Function definition has two major components:
1. Function declarator:
Function declarator is the first line of function definition. When a function is invoked from calling function, control of the program is transferred to function declarator or called function.Syntax of function declarator
return_type function_name(type(1) argument(1),....,type(n) argument(n))Syntax of function declaration and declarator are almost same except, there is no semicolon at the end of declarator and function declarator is followed by function body.
In above example, " int add(int a,int b)" in line 12 is function declarator.
2. Function body:
Function declarator is followed by body of function which is composed of statements.In above example, line 13-19 represents body of a function.
Passing arguments to functions
In programming, argument/parameter is a piece of data(constant or variable) passed from a program to the function.In above example two variable, num1 and num2 are passed to function during function call and these arguments are accepted by arguments a and b in function definition.

Arguments that are passed in function call and arguments that are accepted in function definition should have same data type. For example:
If argument num1 was of int type and num2 was of float type then, argument a should be of int type and b should be of float type in function definition. i.e. type of argument during function call and function definition should be same.
A function can be called with or without an argument.
Return Statement:
Return statement is used for returning a value from function definition to calling function.Syntax of return statement
return (expression;
OR
return;
For example:return;
return a;
return (a+b);
In above example of source code, add is returned (line 18) to the calling function add(num1,num2); (line 8) and this value is stored in variable sum.
Note that, data type of add is int and also the return type of function is int. The data type of expression in return statement should also match the return type of function.
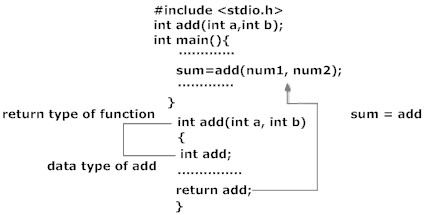
No comments:
Post a Comment